Send an email with an attachment in PHP via AWS SES
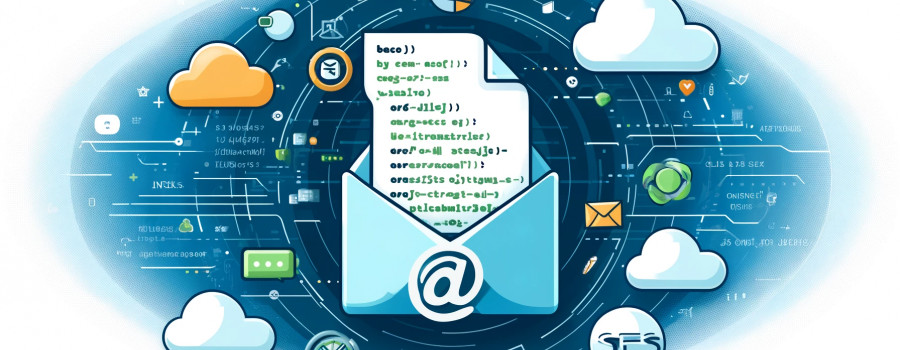
Sending emails is a common task for many websites and applications, and there are several ways to go about it. One option is to use a service such as Amazon's Simple Email Service (SES). In this article, we will show you how to use PHP to send an email with an attachment via SES. We will also discuss some of the benefits of using a service like SES to send your emails, rather than trying to do it yourself.
Here is an example function that sends an email with an attachment via AWS SES in PHP:
function sendEmailWithAttachment($to, $subject, $body, $attachment) {
$client = new SesClient([
'version' => 'latest',
'region' => 'us-east-1',
'credentials' => [
'key' => 'AWS_ACCESS_KEY',
'secret' => 'AWS_SECRET_KEY',
]
]);
try {
$result = $client->sendEmail([
'Destination' => [
'ToAddresses' => [$to],
],
'Message' => [
'Body' => [
'Text' => [
'Charset' => 'UTF-8',
'Data' => $body,
],
],
'Subject' => [
'Charset' => 'UTF-8',
'Data' => $subject,
],
],
'Source' => 'sender@example.com',
'Attachments' => [
[
'Filename' => $attachment['filename'],
'Content' => base64_encode($attachment['data']),
'ContentType' => $attachment['mimeType']
],
],
]);
$messageId = $result['MessageId'];
return true;
} catch (SesException $error) {
return false;
}
}
This function uses the AWS SDK for PHP to send an email via SES (Simple Email Service). It takes four arguments:
- $to: The email address of the recipient
- $subject: The subject of the email
- $body: The body of the email
- $attachment: An array containing the attachment details, including the filename, data, and mimeType of the attachment
The function creates an instance of the SesClient class, which is part of the AWS SDK for PHP and allows you to send emails using SES. It then calls the sendEmail method on this client, passing in the necessary parameters to specify the recipient, subject, body, sender, and attachment of the email. If the email is sent successfully, the function returns true, otherwise it returns false.
There are several reasons why you might want to use a service such as SES to send emails, rather than trying to do it yourself:
Reliability: SES is a highly reliable and scalable service that can send a large number of emails without fail. This is especially important if you are sending emails as part of a critical business process.
Deliverability: SES has a reputation for high deliverability, which means that a greater percentage of your emails are likely to end up in the recipient's inbox rather than their spam folder.
Reputation: SES maintains a good reputation with email providers, which can further improve the deliverability of your emails.
Features: SES provides a number of features that can be useful when sending emails, such as the ability to track opens and clicks, customize the "From" address, and set up email sending domains and identities.